CPSC 124, Fall 2011
Sample Answers for Test #1
Question 1. Explain briefly what is meant by high-level programming language and by machine language, and explain the difference between them.
Answer.
Machine language is the "native language" of a computer. A machine language program consists of instructions that are encoded as binary numbers. These instructions can be executed directly by the computer. People don't write programs in machine language. Instead, they write in high-level languages such as Java. High-level language is closer to English and is more structured than machine language. However, before a high-level language program can be executed by a computer, it must first be translated into machine language.
Question 2. What is meant by comments in a computer program? What are the two types of comment in Java? And why is it important to have good comments in a program?
Answer. Comments are text that is added to a program solely for the use of human readers. Comments are completely ignored by the computer. Comments are important to help the reader understand the structure and logic of the program and what it is meant to do. Java has two types of comments: comments which begin with // and end at the end of the line; and comments that begin with /* and end with */. The latter type of comment can extend over more than one line.
Question 3. A novice programmer tries to write a Java code segment that prints out the numbers 0,1,2,3,4,5. The programmer writes the following code, which contains a syntax error and two semantic errors (bugs):
int num; for (num = 0; num < 5; num++); { system.out.println(num); }
a)What is the syntax error?
b)Once just the syntax error is fixed, what, exactly, will the code segment output?
c)hat are the two semantic errors, and how can they be fixed?
Answer.
a) "system" should begin with an upper-case "S". [This is a syntax error because the compiler will see the error and refuse to compile the program.]
b) It prints out the single number 5. [The for only contains an empty statement, so the loop does not do any output. The loop ends when n=5. Then the computer goes on to the output statement statement, which outputs the value of n.]
c) The ";" at the end of the second line has to be removed. This semicolon ends the for loop, so that the output statment is not really in the loop at all. Once the ";" is removed, the loop will print the numbers 0, 1, 2, 3, and 4. It does not print 5, since the loop only continues as long as n<5. To make it include 5 in the output, change num<5 to num<=5 (or to num<6).
Question 4. Some short answer questions...
a)If s is a String variable whose value is "eschew", what is the value of s.charAt(3) ?
b)What are the possible values of the expression: 5 + (int)( 7 * Math.random() )
c)I x is a double variable whose value is 17.4578, show the exact output from the statement
System.out.printf("The answer is %1.3f", x);
Answer.
a) The value is the char 'h'. [The value is character number 3 in the work "eschew", but positions are numbered starting from 0, not from 1.]
b) 5, 6, 7, 8, 9, 10, and 11. [(int)(7*Math.random()) takes on one of the values 0, 1, 2, 3, 4, 5, and 6. Then 5 is added to that value.]
c) The output is: The answer is 17.458 [This is a printf statement. The value of x is substituted for the %1.3f in the format string. The "3" in the format says that there should be 3 digits after the decimal point. When 17.4578 is rounded to three digits after the decimal, the result is 17.458.]
Question 5. According to the Pythagorean Theorem, if the two sides of a right triangle have lengths a and b, then the length of the hypotenuse is the square root of a*a+b*b. Write a complete Java program (starting with public class) that does the following: Ask the user to enter the lengths of the two sides of a right triangle. Compute the length of the hypotenuse. Tell the user the answer. You can use TextIO to read the input. (The program does not need comments.)
Answer.
public class Hypotenuse { public static void main(String[] args) { double a, b; // the lengths of the sides double hypotenuse; System.out.print("What is the first side? "); a = TextIO.getlnDouble(); System.out.print("What is the second side? "); b = TextIO.getlnDouble(); hypotenuse = Math.sqrt( a*a + b*b ); System.out.println("The hypotenuse is " + hypotenuse); } }
Question 6. Let's say that a temperature in the range 65 to 80 is "comfortable." A temperature below 65 is "cold." And a temperature above 80 is "hot." Suppose that temp is a variable of type int whose value has already been set to the current temperature. Write a code segment that outputs "cold," "hot," or "comfortable," depending on the value of temp.
Answer.
if ( temp < 65 ) { System.out.println( "cold" ); } else if ( temp <= 80 ) { System.out.println( "comfortable" ); } else { System.out.println( "hot" ); }
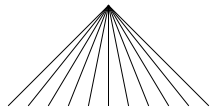
Question 7. Complete the following paintComponent subroutine so that it will draw the picture shown at the right. Use a for loop. There are exactly 11 lines in the picture. The scale that you use is up to you, but your picture should have the same form as the one that is shown. (You do not have to draw fill the background or set the drawing color. Just draw the lines.)
public void paintComponent(Graphics g) { }
Answer.
public void paintComponent(Graphics g) { int i; int x; x = 0; for ( int i = 0; i <= 10; i++ ) { g.drawLine( 100, 0, x, 100 ); x = x + 20; } }
[This assumes that the bottom endpoints of the lines are 20 pixels apart, and that they start at x=0. That puts the last endpoint at x=200. The top point has an x-coordinate that is halfway between 0 and 200, which is 100. I put the y-coord for the top point at 0 and for the bottom points at 100. Each line starts at the top point, (100,0), and goes to one of the bottom points (x,100). The value of x has to increase by 20 between one line and the next. There are many other ways to write this!]
Question 8. Show the exact output produced by the following Java code segment:
int a, b, c; a = 1; b = 2; c = 6; while (c > 0) { if ( c % 2 == 1 ) { a = a * b; } b = b * b; c = c / 2; System.out.println( a + "," + b + "," + c); }
Answer.
1,4,3 4,16,1 64,256,0
[Each time the loop runs, it produces one line of output. The numbers on the line are a, b, and c, separated by commas. If we trace the program, the values of these variables are as follows:
a | b | c ----------------- 1 | 2 | 6 (before the loop starts) 1 | 4 | 3 (the first line of output) 4 | 16 | 1 (the second line) 64 | 256 | 0 (the third line)
Then, since c has become equal to 0, the loop ends.]
Question 9. Write a code segment that does the following: Use a while loop to simulate rolling a pair of dice over and over. Stop when the total showing on the two dice is 12. Count the number of times that the dice are rolled. After the loop, output the count.
Answer.
int count; count = 0; while (true) { int die1, die2; int roll; count++; die1 = (int)(1 + 6*Math.random()); die2 = (int)(1 + 6*Math.random()); roll = die1 + die2; if (roll == 12) { break; } } System.out.println("The number of rolls to get a 12 was " + count);
Or, for another solution,
int die1, die2, roll; int count; count = 0; roll = 0; // to "prime the loop" die1 = (int)(1 + 6*Math.random()); die2 = (int)(1 + 6*Math.random()); roll = die1 + die2; count = count + 1; } System.out.println("The number of rolls to get a 12 was " + count);
Question 10. Write a short essay that discusses the idea of types in Java. What is meant by the type of a variable? How are types used in declaration statements? What do they have to do with assignment statements?
Answer. A type specifies some particular king of data, such as strings of characters, 32-bit integers, or true/false values. Types in Java have names such as String, int, and boolean. A variable in Java represents a location in memory where some data can be stored. The type of a variable determines what kind of data can be stored in that variable. Java is a strongly typed language, which means that a variable can only hold one type of data. That type is specified when the variable is "declared." For example, the variable declaration statements
int k; String name;
say that k is to be a variable that can only hold 32-bit integers, and name is a variable that can only hold strings of characters.
Memory can really hold only binary numbers, 0's and 1's. Binary numbers can encode any kind of value, but in order to interpret a binary number correctly, the computer has to know what type of value it represents. So, the type of a variable also tells the computer how to interpret the 0's and 1's that are stored in that variable.
When a value is assigned to a variable in an assignment statement, the type of the value must match the type of the variable. That is, either the types must be the same, or the type of the value must be one that can be automatically converted into the type of the variable. For example, it is legal to assign and int value to a double variable, because 32-bit integers can be automatically converted into real numbers (with no loss of information). However, it is not legal to assign a double to an int. In some cases, it is possible to use type-casting to force a conversion that would not be done automatically. For example, if x is a variable of type double, then the type-cast (int)x converts the value of x into an int value. To do this, it must drop the fractional part of the value, which involves a real loss of information.