CS 124, Spring 2013
Lab 2: User Input (And Some Graphics)
In the first lab, the only things you could use were assignment statements and output statements. The result could only be a simple program that does the same thing every time it is run. In this lab, we add the capability to get input from the user. With that, what the program does can depend on what the user types into the program when the program is run.
As a bonus, this lab also introduces Java graphics. You'll learn the basic drawing subroutines, and you will use them to draw a simple picture.
To start the lab, you should create a new directory named lab2, inside the cs124 directory that you are using for this course. Please use the name "lab2" exactly, with lower case "l" and no space in the name; this will make it possible for me to find your work more easily.
To do the lab, you will need two files that I have written for you. Copy the files TextIO.java and FirstGraphics.java from the directory /classes/cs124 into your lab2 directory. You can do this using the GUI, or you can do it on the command line by typing the following commands in a new Terminal window:
cd cs124 mkdir lab2 cd lab2 cp /classes/cs124/TextIO.java . cp /classes/cs124/FirstGraphics.java .
(Note that there is a space and a period at the end of the two cp commands. A period is a way of referring to "the current directory." These commands copy files into the current directory, that is, into your lab2 directory in this case.)
Your work from this lab is due at the beginning of next week's lab. When you have finished this lab, you should copy your entire lab2 folder into your homework directory in /classes/cs124/homework. One way to do that is with a command like
cp -r lab2 /classes/cs124/homework/your-last-name
while working in your cs124 directory. Make sure that your solutions to all five exercises are in the lab2 folder that you submit.
Some Style Rules
Starting with this week's lab, part of your grade will be based on program style. When you write a program, it's important to follow rules of good programming style. These rules are mostly for human readers, not for the computer, and therefore the computer won't give you any feedback about them when you compile or run the program. So it's up to you to make sure that your programs show good style. Here are some of the rules that I expect all of your programs to follow:
- Use indentation to show the structure of the program. For example, indent the main routine inside the class, and indent the statements inside the main routine. Each level of indentation should be 3 or 4 spaces. The statements inside the main routine should all line up evenly.
- Use spaces and blank lines to make the program easier on the eyes. For example, it's a good idea to always put spaces around the "=" in an assignment statement. And it's a good idea to put blank lines before and after the main routine, and possibly between sections of the main routing that perform different tasks.
- You should declare just one variable per line (or, in some cases two or three closely related variables).
- In general, every variable declaration should have a comment to explain the purpose of the variable in the program. These are generally one-line comments, starting with //, on the same line as the variable declaration. These comments should describe the purpose of the variable or how it is used in the program. A comment such as "// declare a variable of type int" is worse than useless, since I can tell that much just from reading the Java code.
- Every variable name should begin with a lower case letter. If the name consists of
several words, each word after the first should be capitalized. For example:
myInterestRate
. - Every class name, such as the name of the program, should begin with an upper case letter.
If the name consists of several words, each word should be capitalized. For example:
InterestCalculator
. - Names of variables and classes should be meaningful whenever possible.
InterestCalculator
andmyInterestRate
are meaningful names.MyClass
,IC
, andx
are not. - Use appropriate types. For example, a variable that is naturally an integer should have
type
int
, not typedouble
. - There should be a multi-line comment for the program as a whole that explains the purpose of
the class and, when appropriate, how to use it. This comment goes at the beginning of the java
file, just before
public class...
. - When doing input/output, you should generally prompt the user for inputs, and you should label the outputs. The user must be able to understand what is going on. Include appropriate spaces in your prompts and lables. For example, an output such as "The answer is27" should really be "The answer is 27".
Assignment 1: Testing TextIO
For the time being in this class, you will use TextIO for input.
TextIO
is discussed in
Section 2.4 of the textbook.
TextIO
is not a standard part of Java, so you have to make
it available to the programs in which you want to use it. This means that
TextIO.java
(or at least TextIO.class
) should be
in the same directory with your program. If you followed the directions at
the start of this lab, you have already copied TextIO.java
into your lab2 directory, so any program that you put in that
directory can use all the input subroutines that are defined in the
TextIO
class.
For your first assignment, to make sure that you have TextIO properly working in your lab2 directory, write the Fahrenheit-to-Celsius program that we used as an example in class on Wednesday:
/** * This program reads a temperature measured in degrees Fahrenheit. * It converts the temperature to degrees Celsius and prints the result. */ public class TempConvert { public static void main(String[] args) { double fahrenheit; // The temperature in degrees Fahrenheit. double celsius; // The same temperature in degrees Celsius. System.out.print("What is the temperature in Fahrenheit? "); fahrenheit = TextIO.getlnDouble(); celsius = (fahrenheit - 32) * 5.0/9.0; System.out.printf("The temperature in degrees Celsius is %1.2f", celsius); System.out.println(); } }
(Don't forget that you can simply cut and paste this code from a web browser into a text editor window; you don't have to retype it!)
Compile and run the program to see how it works. Test what happens if
you type in a value that is not a legal real number. Use an "ls"
command on the command line to list the contents of the lab2 folder,
and note that there is a file named TextIO.class
. What is
it, and why do you suppose it was created?
Assignment 2: Basic Arithmetic
In Java, there are five arithmetic operations that you can apply to a pair of integers: +, -, *, /, and %, representing the sum, difference, product, quotient, and remainder of the two numbers. For your second exercise, write a complete Java program that applies each of the five operations to two integers entered by the user. A typical run of the program should look like this, where the user's input is underlined:
Enter your first number: 187 Enter your second number: 23 The sum is 210 The difference is 164 The product is 4301 The quotient is 8 The remainder is 3
Assignment 3: Basic String Manipulation
One important data type in java is String. A String is actually an object, which means that it can contain subroutines. Some of the important subroutine are listed in Subection 2.3.2 in the textbook. One of the most important subroutines in a string str is str.charAt(i), which returns the i-th char in string. For example, if s is a String variable whose value is "Goodbye World:", then s.charAt(0) is 'G', s.charAt(1) is 'o', and so on.
For the third exercise, write a complete program that asks the user to enter their first name and their last name. The program should then print the user's full name and the user's initials. A typical run of the program should look like this, where the user's input is underlined:
What is your first name? David What is your last name? Eck Your full name is David Eck Your initials are DE
Assignment 4: Conversation With Your computer
You should design and write a program that will hold a conversation with the user. The topic and the structure of the conversation is up to you. Requirements are: You should ask the user at least five questions (and read the user's responses). Some of the responses should be numbers, and you should do at least two calculations using the input numbers. (For example, you could ask what year the user was born and have the computer compute the user's age.) Some of the responses should be strings, such as the user's name or favorite color. You should use the user's inputs somehow in your outputs. (For example, "How about that, Fred, blue is my favorite color too.")
Your grade will be partly based on how natural and interesting the conversation is. Feel free to be amusing, obnoxious, philosophical, or whatever. Don't forget that you have to do some computation with the user's input! A conversation might do something like this:
Hi, my name is Kirk. What's your name? Spock Gee, Spock is a nice name. Say, how many years old are you? 187 Let's see, this is the year 2471, so you must have been born in 2284. That was a good year. . . .
But you're not required to duplicate this conversation. the assignment is to make up your own.
Assignment 5: Introduction to Java Graphics
In the last part of the lab, you will get your first taste of GUI programming. Right now, all you can write is a "once-through" list of instructions that is executed from beginning to end -- but you can do anything with those instructions, as long as you have access to the right subroutines. In the previous exercises, you used command-line input and ouput subroutines. In this part of the lab, you will use some of Java's built-in drawing subroutines.
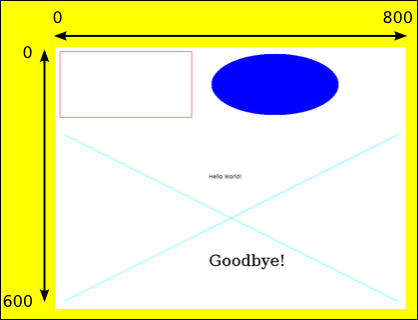
There is a lot about GUI programming that you won't understand for
a while, such as how to open windows and respond to the user's actions.
But as far as actually drawing on the screen, it all comes down to a
bunch of subroutines for drawing basic geometric shapes. The
program FirstGraphics.java has a very simple example of this.
You can compile and run the program to see what it does.
The main()
routine of this program creates a window
and puts it on the screen; you don't have to understand how that part is done!
The actual drawing is done in another routine, which is named
paintComponent()
. The paintComponent routine
contains a list of subroutine call statements. This list of instructions
draws the picture. To draw a different picture,
you just have to substitute your own list of instructions.
Drawing uses (x,y) coordinates, where x goes from 0 on the left to some maximum value at the right, and y goes from 0 at the top to some maximum value at the bottom. The coordinates for this type of graphics must be integers, not real numbers. (The maximum values for x and y are 800 and 600 in this program. You can probably guess how to change these values if you look at the main() routine.) The illustration shows the picture that is drawn when FirstGraphics is run. The picture is in the white area. The numbers and arrows show how the coordinates work. Of course, you can compile and run the program to see the picture at full size.
Here is the original printComponent
routine from that program. You
should try to understand how it works, after reading the descriptions of
the subroutines given below. Make sure that you understand how the
coordinates are being used and how these commands produce the picture
shown above! You will use a lot of similar commands for your own drawing.
protected void paintComponent(Graphics g) { g.setColor(Color.WHITE); // Set color to be used for SUBSEQUENT drawing. g.fillRect(0, 0, 800, 600); // Fill the drawing area with white. g.setColor(Color.RED); g.drawRect(10, 10, 300, 150); // Draw the outline of a rectangle. g.setColor(Color.BLUE); g.fillOval(355, 15, 290, 140); // Draw a filled-in oval. g.setColor(Color.CYAN); g.drawLine(20, 200, 780, 580); // Draw two lines to make a big "X". g.drawLine(20, 580, 780, 200); g.setColor(Color.BLACK); g.drawString("Hello World!", 350, 300); // Draw a string in default font. g.setFont(new Font("Serif", Font.PLAIN, 36)); g.drawString("Goodbye!", 350, 500); // Draw a string using a bigger font. }
For the final assignment of the lab, you should
modify FirstGraphics.java
to draw a different picture.
Erase everything inside the printComponent
routine, and
replace it with your own code. Try to make a picture of some recognizable object, such as
a house, a boat, a face, or a snowman. Maybe use a multicolor background
(representing ground and sky). Add some detail, like clouds or
stars. At a minimum, your picture should use at least a dozen drawing commands and
several different colors, and you should draw at least one String (maybe
containing a title for your picture). Grade will be based partly on
ambition and execution (and maybe a little bit on artistic merit, though
I'm not really the one to judge that).
The following subroutines are available for you to use in the
paintComponent
routine:
g.drawRect(x,y,w,h)
-- Draws the outline of a rectangle whose upper left corner is at the point(x,y)
. The width and height of the rectangle are specified byw
andh
.g.fillRect(x,y,w,h)
-- Draws the same rectangle asg.drawRect(x,y,w,h)
, but filled in with color instead of just outlined.g.drawOval(x,y,w,h)
-- Draws the outline of the oval that just fits inside the rectangle that would be drawn byg.drawRect(x,y,w,h)
. For a circle, w and h should be the same number.g.fillOval(x,y,w,h)
-- Draws a filled-in oval.g.drawString(str,x,y)
-- The first parameter,str
, must be a string; this string is drawn into the picture. The left endpoint of the baseline of the string is at the point(x,y)
. (The baseline is the line on which the string is drawn; the bottoms of letters such as "y" and "g" extend below the baseline.)g.setColor(c)
-- Changes the color that will be used for drawing. The new color applies to drawing commands that follow thesetColor
commands, up until the nextsetColor
command.g.setFont(f)
-- Specifies that the computer should use the fontf
for subsequent calls tog.drawString
. The font determines the size and style of the characters that are drawn bydrawString
.
Predefined colors for use as the "c" value in g.setColor(c)
include:
Color.BLACK
, Color.WHITE
, Color.GRAY
,
Color.LIGHT_GRAY
, Color.DARK_GRAY
, Color.RED
,
Color.GREEN
, Color.BLUE
, Color.YELLOW
,
Color.CYAN
, Color.MAGNETA
,
Color.ORANGE
, and Color.PINK
.
(If you want to know how to make other colors, ask about it.) The sample
program in FirstGraphics.java has an example of using g.setFont(f)
to change the font. If you want to know what is going on there, ask.