CS424 Notes, 3 February 2012
- Discussion
- We will spend part of the class discussing...
- Possible topics for the reserach project. (Topic selection
due February 14; report due March 9.) The project and some
possible topics are discussed in the
course handout.
A couple more possibilities: GPU hardware architecture; old-fashioned OpenGL
with glBegin/glEnd; the old OpenGL fixed-function pipeline.
- Some specific examples of using transforms to create hierarchical
models.
- Image Textures
- The term texture in its most general sense refers to a quantity
that varies from point to point within a primitive. Most common in computer
graphics, however, are image textures.
- When an image is used as a texture, the image is "applied" to a surface.
In general, this means stretch, shrinking, and distorting the image -- especially
for curved shapes in 3D. Here, for example is a picture of my WebGL logo
applied to a teapot model. Multiple copies of the picture are used, with
varying degrees of distortion:
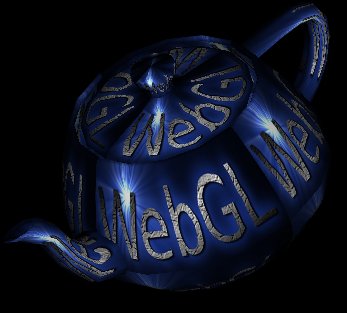
(The teapot is a standard model for 3D graphics testing.)
- Texture coordinates
- The most basic thing to understand is how the mapping of the image
onto a surface is specified. (This actually applies to all textures,
not just image textures.) The mapping is specified by texture coordinates.
- First of all, a coordinate system is applied to the texture image
in which the lower left corner is (0,0) and the upper right is (1,1).
The coordinates are usually referred to as s and t, so
s ranges from 0.0 on the left edge of the image to 1.0 on the
right, and t ranges from 0.0 on the bottom edge to 1.0 at the top.
Note that the 0 to 1 ranges are always used, even if the image is not
square!
- To apply the texture image to a triangle, you have to say what point
in the image maps to each vertex of the triangle. The (s,t) coordinates
that you assign to a vertex are called the texture coordinates
for that vertex. (Texture coordinates for the pixels inside the primitive
are computed by interpolating the texture coordinates that are specified
for the vertices.) For example, suppose you want to map the region outlined
in orange in the following image to a triangular primitive:
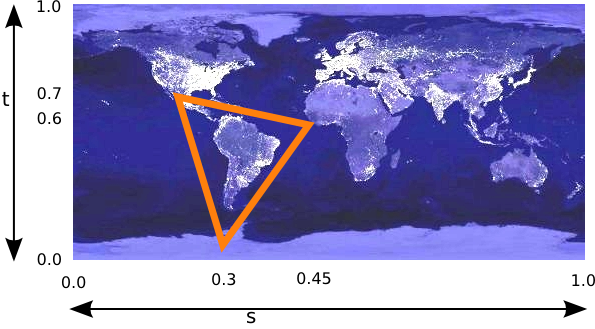
You would do this by specifying the following texture coordinates for
the three vertices of the primitive: (0.3,0.05), (0.45,0.6), and (0.25,0.7).
The triangle would then contain a picture of South America. The South
America on the primitive might have to be stretched and distorted to match
that particular primitive's shape.
- It's possible to provide texture coordinates that are outside the
range 0 to 1. There are several ways to interpret such coordinates, and
what is actually done with them depends on a setting in OpenGL. The
most common interpretation is to imagine that the texture image is
repeated horizontally and vertically to fill the entire st-plane.
For example, the coordinates (3.75,1.2) would be equivalent to (0.75,0.2).
(Unfortunately, this is not the OpenGL default.)
- Other notes...
- The pixels in a texture image are usually referred to as texels.
- Texture images for WebGL should have a width and height that are powers of
2. Common sizes are 64, 128, 256, 512, and maybe 1024. You don't want them
to be too big. While this is not an absolute requirement, non-power-of-two
textures have certain limitations, and it's best to avoid them.
- One nice thing in WebGL is that you can use HTML Image objects
as texture images. We'll see how to do this later.
- When texturing a primitive in OpenGL, you will want to provide one
pair of (s,t) coordinates for each vertex of the primitive. The natural
way to do this is to have an attribute variable in the vertex shader to
represent the texture coordinates. Unfortunately, there's a lot more
to texturing than just providing texture coordinates.
- Textures don't have to come from images. For example, it is also
possible to simply compute the color for each pixel, depending on
its texture coordinates. This is called a procedural texture.
We will see some examples of this soon.